这次不是直接讲解下去,而是谈一下如何把我们写的游戏做成一个exe文件,这样一来,用户不需要安装python就可以玩了。扫清了游戏发布一大障碍啊!
perl,python,java等编程语言,非常好用,语法优美,功能强大;VB啥的,功能上编写的时候总有那么点不舒服的地方(个人见解),可是用户和受众极多,一个很大的原因就是:VB是微软提供的,可以很方便的编译(伪?)生成exe文件。有了exe,所有的Windows都能方便的使用了。
我们不能指望用户在玩我们的游戏之前都安装一个python和pygame,甚至还要装一些其他额外的库(比如上一章的gameobjects),这会吓退99%以上的人……所以把我们的游戏打包(注意是打包而不是编译,python毕竟是脚本程序)成一个可执行文件势在必行。
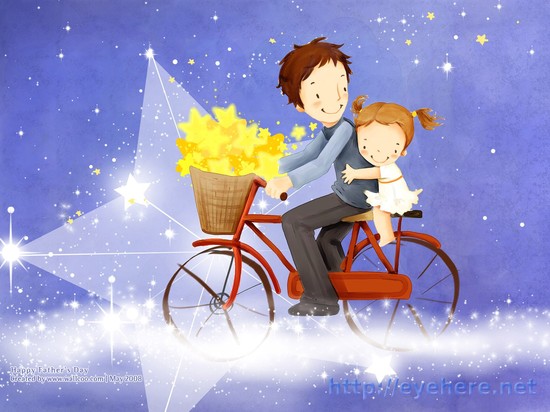
今天是父亲节啊,祝天下父亲快乐平安~
perl有perlcc(免费高效但配置极其复杂),perlapp(简单效果也不错但是收费)等工具;而对python来说,py2exe是不二之选,首先是免费的,而且压出来的文件,虽然不能和编译软件相比,还是不错的了。
到py2exe的官方网站下载安装包,注意要对应自己的python版本。
py2exe是需要写一个脚本进行打包的操作,使用下面这个专为pygame写就的脚本(参考py2exe官方),可以极大的方便打包操作,注意在使用前修改BuildExe里的各个参数。
#!python
# -*- coding: gb2312 -*-
# 这个脚本专为pygame优化,使用py2exe打包代码和资源至dist目录
#
# 使用中若有问题,可以留言至:
# //eyehere.net/2011/python-pygame-novice-professional-py2exe/
#
# 安装需求:
# python, pygame, py2exe 都应该装上
# 使用方法:
# 1: 修改此文件,指定需要打包的.py和对应数据
# 2: python pygame2exe.py
# 3: 在dist文件夹中,enjoy it~
try:
from distutils.core import setup
import py2exe, pygame
from modulefinder import Module
import glob, fnmatch
import sys, os, shutil
except ImportError, message:
raise SystemExit, "Sorry, you must install py2exe, pygame. %s" % message
# 这个函数是用来判断DLL是否是系统提供的(是的话就不用打包)
origIsSystemDLL = py2exe.build_exe.isSystemDLL
def isSystemDLL(pathname):
# 需要hack一下,freetype和ogg的dll并不是系统DLL
if os.path.basename(pathname).lower() in ("libfreetype-6.dll", "libogg-0.dll", "sdl_ttf.dll"):
return 0
return origIsSystemDLL(pathname)
# 把Hack过的函数重新写回去
py2exe.build_exe.isSystemDLL = isSystemDLL
# 这个新的类也是一个Hack,使得pygame的默认字体会被拷贝
class pygame2exe(py2exe.build_exe.py2exe):
def copy_extensions(self, extensions):
# 获得pygame默认字体
pygamedir = os.path.split(pygame.base.__file__)[0]
pygame_default_font = os.path.join(pygamedir, pygame.font.get_default_font())
# 加入拷贝文件列表
extensions.append(Module("pygame.font", pygame_default_font))
py2exe.build_exe.py2exe.copy_extensions(self, extensions)
# 这个类是我们真正做事情的部分
class BuildExe:
def __init__(self):
#------------------------------------------------------#
##### 对于一个新的游戏程序,需要修改这里的各个参数 #####
#------------------------------------------------------#
# 起始py文件
self.script = "MyGames.py"
# 游戏名
self.project_name = "MyGames"
# 游戏site
self.project_url = "about:none"
# 游戏版本
self.project_version = "0.0"
# 游戏许可
self.license = "MyGames License"
# 游戏作者
self.author_name = "xishui"
# 联系电邮
self.author_email = "blog@eyehere.net"
# 游戏版权
self.copyright = "Copyright (c) 3000 xishui."
# 游戏描述
self.project_description = "MyGames Description"
# 游戏图标(None的话使用pygame的默认图标)
self.icon_file = None
# 额外需要拷贝的文件、文件夹(图片,音频等)
self.extra_datas = []
# 额外需要的python库名
self.extra_modules = []
# 需要排除的python库
self.exclude_modules = []
# 额外需要排除的dll
self.exclude_dll = ['']
# 需要加入的py文件
self.extra_scripts = []
# 打包Zip文件名(None的话,打包到exe文件中)
self.zipfile_name = None
# 生成文件夹
self.dist_dir ='dist'
def opj(self, *args):
path = os.path.join(*args)
return os.path.normpath(path)
def find_data_files(self, srcdir, *wildcards, **kw):
# 从源文件夹内获取文件
def walk_helper(arg, dirname, files):
# 当然你使用其他的版本控制工具什么的,也可以加进来
if '.svn' in dirname:
return
names = []
lst, wildcards = arg
for wc in wildcards:
wc_name = self.opj(dirname, wc)
for f in files:
filename = self.opj(dirname, f)
if fnmatch.fnmatch(filename, wc_name) and not os.path.isdir(filename):
names.append(filename)
if names:
lst.append( (dirname, names ) )
file_list = []
recursive = kw.get('recursive', True)
if recursive:
os.path.walk(srcdir, walk_helper, (file_list, wildcards))
else:
walk_helper((file_list, wildcards),
srcdir,
[os.path.basename(f) for f in glob.glob(self.opj(srcdir, '*'))])
return file_list
def run(self):
if os.path.isdir(self.dist_dir): # 删除上次的生成结果
shutil.rmtree(self.dist_dir)
# 获得默认图标
if self.icon_file == None:
path = os.path.split(pygame.__file__)[0]
self.icon_file = os.path.join(path, 'pygame.ico')
# 获得需要打包的数据文件
extra_datas = []
for data in self.extra_datas:
if os.path.isdir(data):
extra_datas.extend(self.find_data_files(data, '*'))
else:
extra_datas.append(('.', [data]))
# 开始打包exe
setup(
cmdclass = {'py2exe': pygame2exe},
version = self.project_version,
description = self.project_description,
name = self.project_name,
url = self.project_url,
author = self.author_name,
author_email = self.author_email,
license = self.license,
# 默认生成窗口程序,如果需要生成终端程序(debug阶段),使用:
# console = [{
windows = [{
'script': self.script,
'icon_resources': [(0, self.icon_file)],
'copyright': self.copyright
}],
options = {'py2exe': {'optimize': 2, 'bundle_files': 1,
'compressed': True,
'excludes': self.exclude_modules,
'packages': self.extra_modules,
'dist_dir': self.dist_dir,
'dll_excludes': self.exclude_dll,
'includes': self.extra_scripts} },
zipfile = self.zipfile_name,
data_files = extra_datas,
)
if os.path.isdir('build'): # 清除build文件夹
shutil.rmtree('build')
if __name__ == '__main__':
if len(sys.argv) < 2:
sys.argv.append('py2exe')
BuildExe().run()
raw_input("Finished! Press any key to exit.")
可以先从简单的程序开始,有了一点经验再尝试打包复杂的游戏。
一些Tips:
- 如果执行出错,会生成一个xxx.exe.log,参考这里的log信息看是不是少打包了东西。
- 一开始可以使用console来打包,这样可以在命令行里看到更多的信息。
- 对于每一个游戏,基本都需要拷贝上面的原始代码修改为独一无二的打包执行文件。
- 即使一个很小的py文件,最终生成的exe文件也很大(看安装的库而定,我这里最小4.7M左右),事实上py2exe在打包的时候会把无数的不需要的库都打进来导致最终文件臃肿,如果你安装了很繁杂的库(wxPython等)更是如此。使用zip打包以后查看里面的库文件,把不需要的逐一加入到self.exclude_modules中,最后可以把文件尺寸控制在一个可以接受的范围内。
2011/08/21 追记:
很多人在打包使用Font模块时出现问题,这里需要把sdl_ttf.dll声明为非系统文件,我已经修改了脚本默认就加入了。而且建议,如果将来是确定要打包为exe的,那么就不要使用系统字体,即"pygame.font.SysFont(xxx)",而是使用字体文件,然后打包时将文件当作图片等一起打包,这样出问题的概率会大大降低。
2011/09/24 追记:
感谢blues_city网友,“dist_dir”应该是属于py2exe的特有options而不是setup的。
欢迎大家试用并提出建议,不断完善这个脚本。
这个系列写完了吗?
不,远远没有~
哗,远远没有,,,有大爱,到50时,可以考虑联系出版社了,,,
博主好,我参考了您的setup文件打包我写的pygame的小游戏,编译之后打开exe文件出现错误
MemoryLoadLibrary failed loading pygamefont.pyd
google了一下,有人说不要用单一文件,于是我编辑了BuildExe.zipfile_name打包成zip文件。并声明”sdl_ttf.dll”为非系统文件,重新打包之后执行,报错:
Runtime Error!
This application has requested the Runtime to terminate it in an unusual way.
Please contact the application’s support team for more information.
目前仍未找到解决方案,可以确定的是错误源于读取字体。另外搜索过程中找到这位有类似问题:
http://www.daniweb.com/software-development/python/threads/247249
最后一位回帖者称是版本问题。
为寻求答案来此留言,期待博主抽空提出意见,先谢过
我在遇到这个问题的时候,还是用了打包,仅仅是将”sdl_ttf.dll”加一下就解决了。不介意的话可以把您的代码发给我测试一下(可以把其他都删除,仅保留必要和使用font那部分能够出现问题便可)。另外请告知系统、python、pygame的版本。
大神你好,我用的2.7,转出来的EXE文件打不开,log:
E:\python\bird\dist\bird.exe:17: RuntimeWarning: use mixer: DLL load failed: 找不到指定的模块。
(ImportError: DLL load failed: 找不到指定的模块。)
Traceback (most recent call last):
File “bird.py”, line 17, in
File “pygame\__init__.pyc”, line 70, in __getattr__
NotImplementedError: mixer module not available
(ImportError: DLL load failed: 找不到指定的模块。)
我看了一下源代码第十七行,是pygame.mixer.init(),可是我前面已经import pygame了啊,这是怎么回事
python3.4;win7-64位;pygame1.9.2a0
xishui大神好~~
你的入门到精通系列实在是太棒了~按着教程,我写了个俄罗斯方块,打包exe的时候把上面代码直接复制了,可是打包出的exe不能用……
log信息:
C:Python27distnoname.exezipextimporter.py:82: RuntimeWarning: import display: No module named _view
(ImportError: No module named _view)
C:Python27distnoname.exezipextimporter.py:82: RuntimeWarning: import draw: No module named _view
(ImportError: No module named _view)
C:Python27distnoname.exezipextimporter.py:82: RuntimeWarning: import image: No module named _view
(ImportError: No module named _view)
C:Python27distnoname.exezipextimporter.py:82: RuntimeWarning: import pixelcopy: No module named _view
(ImportError: No module named _view)
C:Python27distnoname.exezipextimporter.py:82: RuntimeWarning: import transform: No module named _view
(ImportError: No module named _view)
Traceback (most recent call last):
File “noname.py”, line 27, in
File “pygame__init__.pyo”, line 70, in __getattr__
NotImplementedError: display module not available
(ImportError: No module named _view)
能帮忙看一下吗?我的py是2.7
万分感谢
太客气了,能让大家学到一点有用的东西再好不过了。
我从没遇到过 _view库丢失的问题…… 有试着先编译一个简单的脚本么?我用的是2.6,虽然应该不至于但并不能确定在2.7下一定是正常的,如果没头绪并且不介意的话,可以把你的代码发给我来测试一下,邮箱在About里。
不知道,博主是怎么解决2.7下, _view库丢失的问题的??希望博主解释一下,因为我也遇到了这个问题!!
row 76: self.extra_modules=[‘pygame._view’]
博主您好,我打包也出现了这个问题,目前还没有解决!求助
博主您好,我打包也出现了这个问题,目前还没有解决!求助
C:\py2exe\dist\MyGames.exe\zipextimporter.py:82: RuntimeWarning: import display: No module named _view
(ImportError: No module named _view)
C:\py2exe\dist\MyGames.exe\zipextimporter.py:82: RuntimeWarning: import draw: No module named _view
(ImportError: No module named _view)
C:\py2exe\dist\MyGames.exe\zipextimporter.py:82: RuntimeWarning: import image: No module named _view
(ImportError: No module named _view)
C:\py2exe\dist\MyGames.exe\zipextimporter.py:82: RuntimeWarning: import pixelcopy: No module named _view
(ImportError: No module named _view)
C:\py2exe\dist\MyGames.exe\zipextimporter.py:82: RuntimeWarning: import transform: No module named _view
(ImportError: No module named _view)
Traceback (most recent call last):
File “MyGames.py”, line 11, in
File “pygame\__init__.pyo”, line 70, in __getattr__
NotImplementedError: display module not available
(ImportError: No module named _view)
真是对不住,我已经很久没有玩Python了,源码等也已经不知去向,没法帮到你了……
@xishui: 我在self.extra_modules里加了”pygame”,就解决了~虽然不知什么原理,不过总算OK了呵呵
main.exe:10: RuntimeWarning: use font: DLL load failed: 找不到指定的模块。
(ImportError: DLL load failed: 找不到指定的模块。)
Traceback (most recent call last):
File “main.py”, line 10, in
File “pygame__init__.pyc”, line 70, in __getattr__
NotImplementedError: font module not available
(ImportError: DLL load failed: 找不到指定的模块。)
我也出现了这个错误,请问下博主是怎么解决的?谢谢~~
试下一个你这个脚本,有几个地方有错呀,虽然并不是什么重要的问题倒是了。
这个设定输出目录有错呀。
dist_dir = self.dist_dir
这个应该是在给py2exe的参数里的
修正办法在 156 - 157 这几行中随便加入一行如下代码。
“dist_dir” : self.dist_dir , #生成目录
Thank you! 一直以来用默认名字dist都没有发现这个问题,马上修改,感谢您的提醒。
@Comix: import pygame._view 就行了
@hanke9087: 还是不行……依然ImportError: DLL load failed:
@hanke9087:
……依然No module named _view
你好 你的pygame._view
最后是怎么解决的?
跪求
又是一年父亲节,在此留个名。博主的博客都很不错,支持ing 🙂
line 27 in
origIsSystemDLL = py2exe.bulid_exe.isSystemDLL
AttributeError: ‘module’ object has no attribute ‘bulid_exe’
报错信息是这个,我百度了找不到解决方法。。
技术问题请勿用百度,尽管Google被百般和谐,还是比度娘好用~ 您有没有觉得build和bulid长得很像?
if len(sys.argv) < 2:
提示我这句语法错误,怎么回事啊
不知为何小于号<被改成了<,估计是上次数据导入导出的时候出了点小差池……
我用的是Win7 64位,python26,pygame是1.9.1的
给入门到精通(8)中的最后一个例子打包时
会有pywintypes26.dll找不到
然后我将这个dll排除就好了……
self.exclude_dll = [‘pywintypes26.dll’]
出现了问题,但是log文件没找到,应该在哪里找?
打包时出错了:SystemExit: error: bundle-files 1 not yet supported on win64这个是什么意思啊?求大神指点
很简单的方法,安装32位的Python和相应的库,有些功能64位的并不支持…… 感觉个人学习,32位完全足够了。
SystemExit: error: command ‘”D:pythonw.exe”‘ failed with exit status 1
我在打包时出现了上述错误,请问应该如何解决?
File “D:my_python_studyfighterpy2exe_dabao.py”, line 32, in isSystemDLL
return origIsSystemDLL(pathname)
File “D:my_python_studyfighterpy2exe_dabao.py”, line 30, in isSystemDLL
if os.path.basename(pathname).lower() in (“libfreetype-6.dll”, “libogg-0.dll”, “sdl_ttf.dll”):
File “F:Program FilesPython25libntpath.py”, line 200, in basename
return split(p)[1]
File “F:Program FilesPython25libntpath.py”, line 164, in split
d, p = splitdrive(p)
File “F:Program FilesPython25libntpath.py”, line 119, in splitdrive
if p[1:2] == ‘:’:
RuntimeError: maximum recursion depth exceeded in cmp
请教一下,打包出现上面错误,这是最后几句的
我只改了self.script这个参数,是哪里不对呢??
如果是仅改了”self.script”,请确定那个文件名是你写的一个脚本,而且那个脚本可以正常运行,如果您确实要递归很多次——虽然我觉得不太有那个可能性——您可以使用sys.setrecursionlimit来暂时增加这个次数限制。
谢谢啦~虽然还是没搞懂。。。。不过。。暂时先不纠结这块了,从您这里确实学到了不少东西,十分感谢~
请教,runtime error!错误如何解决?
游戏需要额外的音频、图片的话,那么self.icon_file = None怎么改呢?
游戏需要额外的音频、图片的话,为何生成的exe无法打开:
Traceback (most recent call last):
File “game.py”, line 22, in
pygame.error: Couldn’t open resources/images/dude.png
难道不支持pygame游戏的打包吗?
请问self.extra_datas里的是只是文件名吗?用不用包含路径?
运行的时候出现:
File “D:pythonlibntpath.py”, line 256, in walk
names = os.listdir(top)
TypeError: coercing to Unicode: need string or buffer, NoneType found
请问下楼主有没有用过pyinstaller 打包pygame 程序。
我试过打包单个py文件,pygame文件打包后执行会闪退。
多谢楼主的辛勤付出。
我的打包出现错误,显示no module named numpy.distutils.tests.
之后我找到numpy\distutils.tests\目录,建立了一个空白的__init__.py文件,仍然无法解决。在exclude_modules中加上它也不行。
后来又尝试用pyinstaller,也是像@done一样的问题,执行闪退。
希望楼主帮助。
update我自己:我在 self.extra_modules 中干脆删除了numpy。运行脚本貌似成功了,但是运行exe的时候出错,打开log文件显示无法打开图片资源文件:
pygame.error: Couldn’t open G:\tdgame_multithreaded\tdgame\dist\missions.exe\objects\image_riflebullet_1x5x1x15.png
其中missions.exe是在zipfile_name设为None之后生成的,如果设为别的什么就相应变成设置的名字,结果仍然不能打开。如果在option中把zipfile去掉,它自动生成一个library.zip文件,但是无法打开它,所以图片文件还是不能读取。总之我猜测应该是这里有问题,但是不知道怎么解决。
update myself。py2exe仍然是什么图片也打不开。不过我在pyinstaller 上成功了!font还是需要自己打包进ttf文件才行。不过至少运行成功,虽然其间运行不太流畅。我觉得这种破烂游戏不应该有什么大运算啊,为什么这么卡呢?
能分享一下吗,让大家瞧瞧慢的罪魁祸首是什么
可以啊。不过慢的问题已经解决了,原来是放了不必要的图形操作进入了主循环,然后内存就满了。
给出我网盘上分享的地址欢迎博主和其他朋友去下载玩玩。
http://pan.baidu.com/s/1dD9p6Pr
谢谢楼主,
可以详细说下打包的步骤吗?
我用你给的那个脚本总报错,no such file or directory
然后我用py2exe.org的那个三行的脚本就转出来exe了。
不知道用它那个少的转pygame可以吗?
受教了 这个东西的确很有用 支持博主有空的时候把那本英文书全部翻译出版一下 我会支持正版滴~ :)
大神你好:我运行你的程序,也改了bulidExe,可是我的dist文件夹里什么都没有
运行时总出现mixer module not available找不到指定模块
Devils jerseys china,No sale tax, 100% satisfaction, worldwide shipping within one week.
official Oilers jersey http://teamcharlottelax.com/video/aznbwk1.html
from distutils.core import setup
import glob
import py2exe
import sys,os
import pygame
from pygame.locals import *
import math
import random
import pygame.mixer
from pygame import mixer
sys.path.append(“D:/Program Files/Python2.7/Lib/site-packages/pygame”)
INCLUDES = [“encodings”, “encodings.*”,”pygame.mixer”,”pygame.font”]
extra_modules = [‘pygame’]
options = {“py2exe” :
{“compressed” : 1,
“optimize” : 2,
“bundle_files” : 2,
“includes” : INCLUDES,
“packages” :extra_modules,
“dll_excludes”: [ “MSVCP71.dll”, “mswsock.dll”, “powrprof.dll”,”w9xpopen.exe”]
}}
setup(
options = options,
windows=[“game.py”],
zipfile = None,
data_files=[(“resources/audio”,
glob.glob(“resources/audio/\*.wav”)),
(“resources/images”, glob.glob(“resources/images/\*.png”))])
Traceback (most recent call last):
File “game.py”, line 7, in
File “zipextimporter.pyo”, line 98, in load_module
ImportError: MemoryLoadLibrary failed loading pygame\mixer.pyd
产生这个错误该怎么解决,查了好多资料都不行。谢谢啊!
xishui楼主你好,感谢你提供的脚本。
我的python游戏已经编译成功了,但是运行时出现
“Fatal Python error: (pygame parachute) Segmentation Fault”
这样的错误,请问您有遇到过类似的问题吗?
代码挂在‘https://github.com/piratf/Mytools/tree/master/myCastle’
一共两百行 有注释,python环境下运行没问题,2.7 求楼主帮忙看下哪里出了问题。。
再次感谢楼主啦
debug后发现是字体出了问题,我使用的是在resources文件夹里单独放的字体文件,还是不能用。
e:\Code\game\myCastle\myCastle.exe:144: RuntimeWarning: use font: MemoryLoadLibrary failed loading pygame\font.pyd
(ImportError: MemoryLoadLibrary failed loading pygame\font.pyd)
Traceback (most recent call last):
File “myCastle.py”, line 144, in
font = pygame.font.SysFont(“Yahei Consolas Hybrid”, 24)
File “pygame\__init__.pyo”, line 70, in __getattr__
NotImplementedError: font module not available
(ImportError: MemoryLoadLibrary failed loading pygame\font.pyd)
请问xishui大大“把sdl_ttf.dll声明为非系统文件”应该怎么做呢?或者还有别的方法吗? 谢谢!
抱歉刚才粘贴的是系统字体出问题的内容。。 单独字体文件也是不行
后来用pyinstall编译过了,还是不知道为什么这个脚本过不了。不过还是谢谢楼主的脚本!希望有空一起交流,谢谢!
———- python ———-
running py2exe
creating D:\python\Mydoc\PYGAME\PyGame\build
creating D:\python\Mydoc\PYGAME\PyGame\build\bdist.win32
creating D:\python\Mydoc\PYGAME\PyGame\build\bdist.win32\winexe
creating D:\python\Mydoc\PYGAME\PyGame\build\bdist.win32\winexe\collect-2.7
creating D:\python\Mydoc\PYGAME\PyGame\build\bdist.win32\winexe\bundle-2.7
creating D:\python\Mydoc\PYGAME\PyGame\build\bdist.win32\winexe\temp
creating D:\python\Mydoc\PYGAME\PyGame\dist
*** searching for required modules ***
Traceback (most recent call last):
File “game_to_exe.py”, line 172, in
BuildExe().run()
File “game_to_exe.py”, line 163, in run
data_files = extra_datas,
File “D:\python\lib\distutils\core.py”, line 151, in setup
dist.run_commands()
File “D:\python\lib\distutils\dist.py”, line 953, in run_commands
self.run_command(cmd)
File “D:\python\lib\distutils\dist.py”, line 972, in run_command
cmd_obj.run()
File “D:\python\lib\site-packages\py2exe\build_exe.py”, line 243, in run
self._run()
File “D:\python\lib\site-packages\py2exe\build_exe.py”, line 296, in _run
self.find_needed_modules(mf, required_files, required_modules)
File “D:\python\lib\site-packages\py2exe\build_exe.py”, line 1306, in find_needed_modules
mf.import_hook(f)
File “D:\python\lib\site-packages\py2exe\mf.py”, line 719, in import_hook
return Base.import_hook(self,name,caller,fromlist,level)
File “D:\python\lib\site-packages\py2exe\mf.py”, line 136, in import_hook
q, tail = self.find_head_package(parent, name)
File “D:\python\lib\site-packages\py2exe\mf.py”, line 181, in find_head_package
if ‘.’ in name:
TypeError: argument of type ‘module’ is not iterable
输出完毕 (耗时 27 秒) – 正常终止
这种错误怎么解决啊?
Pingback: 用Python和Pygame写游戏-从入门到精通(py2exe篇)-演道网
py2exe 好像没有python3的版本
现在有了,支持到py3.4
感谢博主,学到了很多xd
不过话说这个脚本怎么换图标icon?
我怎么写都不对,直接self.icon_file = “icon.png”也是错的
大神你好,我运行后总是有这样的错误AttributeError: ‘module’ object has no attribute ‘build_exe’
python3.6使用pyinstaller发布:
首先,安装pyinstaller,直接pip3 install pyinstaller即可。
然后按以下步骤:
1、需要在py中添加函数:
# 用来加载图片的函数,后续所有需要用到图片的地方,都通过该函数来加载。
def resource_path(relative):
if hasattr(sys, “_MEIPASS”):
return os.path.join(sys._MEIPASS, relative)
return os.path.join(relative)
2、需要加载图片的地方,以前直接用的路径,现在都使用:
resource_path(os.path.join(data_dir, ‘ant.png’))
PS:data_dir是指和脚本同级的用来存放图片的路径,一般使用images。
3、执行pyi-makespec 参数 要打包的py脚本
如:pyi-makespec -F game1.py
4、修改生成的xxx.spec文件
指定要添加的图片:
a.datas += [(‘images\\ant.png’,”D:\\PyCharm\\PycharmProjects\\pygame\\images\\ant.png”,’DATA’),(‘images\\leaf.png’,”D:\\PyCharm\\PycharmProjects\\pygame\\images\\leaf.png”,’DATA’),(‘images\\spider.png’,”D:\\PyCharm\\PycharmProjects\\pygame\\images\\spider.png”,’DATA’)]
如果有多个图片,则可以考虑使用:
dict_tree = Tree(“D:\PyCharm\PycharmProjects\pygame\images”, prefix = “images”)
a.datas += dict_tree
说明:Tree(“实际存放图片的文件夹”,prefix = “代码中图片文件的上级目录。”)
比如说,在代码中希望load(’images/aaa.jpg’),aaa.jpg在本地实际存储路径是:d:\images\
则Tree(“d:\\images”,prefix = “images”)
5、执行pyinstaller xxx.spec
你好 你的pygame._view
最后是怎么解决的?
跪求
Доброго времени суток,спешу поделиться очень важной информацией при выборе детских товаров: магазин для малышей и мам так что, даже если приобретённый дресс-код испачкался, обыкновенная стирка быстро вернёт его в прежнее состояние: весь товар выполнен из натуральных тканей, где нанесённые рисунки, вышивки и прочие виды декора до последнего остаются на службе.наблюдается это крайне редко, но лучше заранее проверить реакцию малыша на новое приспособление.защитная рама расстегивается по центру, однако не подлежит полному съему, и это немного огорчает.
非常精彩的文章,引人入胜,痛快淋漓。感谢楼主分享。
非常精彩的文章,引人入胜,痛快淋漓。感谢楼主分享。
рабочее зеркало гидры – рабочее зеркало гидры, hydra магазин
купить чита пабг – чит для dota2, заказать сайт
ню снимки обнаженных телок – ню фотки голых натуральных титек, картинки обнаженных больших сисек
确实很长,植物大战僵尸这个坑开了九年,只写了1篇文章
индивидуалки москвы – проститутки москвы проверенные, индивидуалки москвы
hydra вход – hydra вход, hydra зеркало
additional resources https://slotumkasyno.com/bonusy/
博主你好,我是在pychorm上运行的,只有一台,服务器客户端怎么同时打开呀,